addElementsToArray
- Generic technical name
- sourceData
- Type
- SourceData
- newElements
- Type
- Array<ArrayElement>
- Required
- Yes
- mutably
- Type
- boolean (true only)
- Required
- Yes
- targetArray
- Type
- Array<ArrayElement>
- Required
- Yes
- mutably
- Type
- boolean (false only)
- Required
- Yes
- targetArray
- Type
- Array<ArrayElement>
- Required
- Yes
- toStart
- Type
- boolean (true only)
- Required
- Yes
- toEnd
- Type
- boolean (true only)
- Required
- Yes
- toPosition__numerationFrom0
- Type
- number (positive integer)
- Required
- Yes
- toPosition__numerationFrom1
- Type
- number (natural)
- Required
- Yes
Adds the new elements to start, end, or specified position of the indexed array. Both changing of the initial array (mutable adding) and creating a new one based on the initial one before adding the new elements (immutable adding) are available, depending on the corresponding option. In the case of immutable adding, only a new container is created while deep copying of each element is not being executed (and could not be executed for the arbitrary object).
Examples
Adding of one element to the start of array
Mutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
addElementsToArray({
targetArray: sampleArray,
newElements: [ "NEW_ELEMENT-1" ],
toStart: true,
mutably: true
});
console.log(sampleArray);
Adding of element
"NEW_ELEMENT-1"
to the start of the array of
strings.
The mutably
option has been made required
not without reason; the programmer must clearly realize
what he is doing.
In this example, the value true
has been set, so the initial
array will change to:
[ "NEW_ELEMENT-1", "INITIALLY_EXISTED_ELEMENT-1", "INITIALLY_EXISTED_ELEMENT-2" ]
.
ReadonlyArray
because the function will change this
array.
This usage of the function is close to the native method
unshift.
The difference is that addElementsToArray
always returns
the updated array, not the count of its
elements as unshift
does.
However, if the element count of the new array is required, it is possible
to access the length
property of the
returned value without defining a new variable.
In addition, unlike unshift
, it is completely obvious
what addElementsToArray
does; specifically, whether or not adding the
elements will change the initial array.
The input required for addElementsToArray
takes more time than
unshift
; however, the crystal clearness of the code and
its maintainability are much more important than the input of the initial code.
Unfortunately, it does not convince many programmers, especially junior programmers because
human psychology prefers momentary profits over greater future profits.
In the development of web sites and software, such a feature is the cause of code that is
hard to maintain, which makes web sites and software lose time and money.
As for the input speed, modern Integrated Development Environments (IDEs)
solve such problems well.
In particular, for theIntelliJ IDEA family IDEs
theofficial YDEE plugin is supported, and it includestemplates for quick input (so-called "Live templates") , such as the one for addElementsToArray
.
Immutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
const updatedSampleArray: Array<string> = addElementsToArray({
targetArray: experimentalSample,
newElements: [ "NEW_ELEMENT-1" ],
toStart: true,
mutably: false
});
console.log(sampleArray);
console.log(updatedSampleArray);
In the case of immutable adding of the elements, a new
array based on the previous one will be created.
Then, the specified element will be added to the
new one.
This way the initial array sampleArray
will
not mutate while the new one
(updatedSampleArray
) will have the value.
[ "NEW_ELEMENT-1", "INITIALLY_EXISTED_ELEMENT-1", "INITIALLY_EXISTED_ELEMENT-2" ]
.
Such anti-intuitive manipulations are demanded mainly in frontend frameworks, and the developers of
some of them (e. g. React) assign users with the task of creating
new state objects based on outdated ones.
addElementsToArray
allows you to write clearer code, in particular when you
need to add one or more elements to the intermediate positions of the
array.
This makes the code simpler.
However, this function does not create a full ("deep") copy of the array. First of all, it is generally impossible to create a deep copy of an arbitrary object (note: in the ECMAScript languages, the array is the superset of the object). For example, the closures and private fields added in ES2022 could not be copied, thus the function for the deep copying of an arbitrary object could not be developed. In the case of adding elements to the array, it is not critical even in React. However, in the case of changing the properties of an object-type array elements, both a new array itself and the new element based on the previous one need to be created.
Adding of one element to the end of array
Mutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
addElementsToArray({
targetArray: sampleArray,
newElements: [ "NEW_ELEMENT-1" ],
toEnd: true,
mutably: true
});
console.log(sampleArray);
Adding of element
"NEW_ELEMENT-1"
to the end of the array of
strings.
The mutably
option has been made required
not without reason; the programmer must clearly realize
what he is doing.
In this example, the value true
has been set, so the initial
array will change to:
[ "INITIALLY_EXISTED_ELEMENT-1", "INITIALLY_EXISTED_ELEMENT-2", "NEW_ELEMENT-1" ]
.
ReadonlyArray
because the function will change this
array.
This usage of the function is close to the native method
push.
The difference is that addElementsToArray
always returns
the updated array, not the count of its
elements as push
does.
However, if the element count of the new array is required, it is possible
to access the length
property of the
returned value without defining a new variable.
In addition, unlike push
, it is completely obvious
what addElementsToArray
does; specifically, whether or not adding the
elements will change the initial array.
The input required for addElementsToArray
takes more time than
push
; however, the crystal clearness of the code and
its maintainability are much more important than the input of the initial code.
Unfortunately, it does not convince many programmers, especially junior programmers because
human psychology prefers momentary profits over greater future profits.
In the development of web sites and software, such a feature is the cause of code that is
hard to maintain, which makes web sites and software lose time and money.
As for the input speed, modern Integrated Development Environments (IDEs)
solve such problems well.
In particular, for theIntelliJ IDEA family IDEs
theofficial YDEE plugin is supported, and it includestemplates for quick input (so-called "Live templates") , such as the one for addElementsToArray
.
Immutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
const updatedSampleArray: Array<string> = addElementsToArray({
targetArray: experimentalSample,
newElements: [ "NEW_ELEMENT-1" ],
toEnd: true,
mutably: false
});
console.log(sampleArray);
console.log(updatedSampleArray);
In the case of immutable adding of the elements, a new
array based on the previous one will be created.
Then, the specified element will be added to the
new one.
This way the initial array sampleArray
will
not mutate while the new one
(updatedSampleArray
) will have the value.
[ "INITIALLY_EXISTED_ELEMENT-1", "INITIALLY_EXISTED_ELEMENT-2", "NEW_ELEMENT-1" ]
.
Such anti-intuitive manipulations are demanded mainly in frontend frameworks, and the developers of
some of them (e. g. React) assign users with the task of creating
new state objects based on outdated ones.
addElementsToArray
allows you to write clearer code, in particular when you
need to add one or more elements to the intermediate positions of the
array.
This makes the code simpler.
However, this function does not create a full ("deep") copy of the array. First of all, it is generally impossible to create a deep copy of an arbitrary object (note: in the ECMAScript languages, the array is the superset of the object). For example, the closures and private fields added in ES2022 could not be copied, thus the function for the deep copying of an arbitrary object could not be developed. In the case of adding elements to the array, it is not critical even in React. However, in the case of changing the properties of an object-type array elements, both a new array itself and the new element based on the previous one need to be created.
Adding of one element to the specific position of array (numeration from 0)
Mutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
addElementsToArray({
targetArray: experimentalSample,
newElements: [ "NEW_ELEMENT-1" ],
toPosition__numerationFrom0: 1,
mutably: true
});
console.log(sampleArray);
Adding the new
element "NEW_ELEMENT-1"
to 1st position
(counting from 0).
Because the initial array had 2 elements,
the new element will be between them, and the
updated array will be:
[ "INITIALLY_EXISTED_ELEMENT-1", "NEW_ELEMENT-1", "INITIALLY_EXISTED_ELEMENT-2" ]
.
The same thing could be done without libraries by using the native method splice, but:
- This method was designed primarily for deleting elements from the array. If we need to only add a new element without deleting, the 2nd parameter will get in the way. (In such a use case of this method, it must be 0.)
- It is a little hard to memorize because the purpose of each parameter is not obvious. One parameter is the index of the element, another one is the quantity of elements, and then the new elements themself.
Immutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
const updatedSampleArray: Array<string> = addElementsToArray({
targetArray: experimentalSample,
newElements: [ "NEW_ELEMENT-1" ],
toPosition__numerationFrom0: 1,
mutably: false
});
console.log(sampleArray);
console.log(updatedSampleArray);
With mutably: false
the new array will be based on
the initial one, then all manipulation will be executed with the new one.
In this example, after creating the new array, the
element "NEW_ELEMENT-1"
will be added to position 1
with counting from 0.
As a result, the new array will be:
[ "INITIALLY_EXISTED_ELEMENT-1", "NEW_ELEMENT-1", "INITIALLY_EXISTED_ELEMENT-2" ]
.
However, this function does not create a full ("deep") copy of the array. First of all, it is generally impossible to create a deep copy of an arbitrary object (note: in the ECMAScript languages, the array is the superset of the object). For example, the closures and private fields added in ES2022 could not be copied, thus the function for the deep copying of an arbitrary object could not be developed. In the case of adding elements to the array, it is not critical even in React. However, in the case of changing the properties of an object-type array elements, both a new array itself and the new element based on the previous one need to be created.
Until recently, without usage of a third-party library, the same manipulation could not be done with a single expression, so it was required to:
- Create the new array
- Copy the elements to this new array
- Call the
splice
method of the new array
These routines are exactly what addElementsToArray
does when the
mutable
option has the value of false
.
In 2023, the toSpliced
method was been
developed, which does same things as splice
, but it preliminary
creates a new array based on the initial one.
Since the spring of 2023, some popular browsers do not support
the toSpliced
method, and, in Node.js,
this method has been available since version 20.
Anyway, you still need to memorize what the parameters of splice
and toSpliced
mean, so addElementsToArray
is still useful, and removal of this function from the library because of the appearance of
toSpliced
is not planned.
Adding one element to the specific position of an array (numeration from 1)
Although, in programming, counting from 0 is the standard, it frequently
confuses programmers and can be a source of mistakes.
This problem was the motivation for the adding of the toPosition__numerationFrom1
option which could be specified instead of
toPosition__numerationFrom0
.
Mutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
addElementsToArray({
targetArray: experimentalSample,
newElements: [ "NEW_ELEMENT-1" ],
toPosition__numerationFrom0: 2,
mutably: true
});
console.log(sampleArray);
Adding the new
elements "NEW_ELEMENT-1"
and
"NEW_ELEMENT-2"
to 2nd position
(counting from 1).
Because the initial array had 2 elements,
the new elements will be between them, and the
updated array will be:
[ "INITIALLY_EXISTED_ELEMENT-1", "NEW_ELEMENT-1", "NEW_ELEMENT-2", "INITIALLY_EXISTED_ELEMENT-2" ]
.
The same thing could be done without libraries by using the native method splice, but:
- This method was designed primarily for deleting elements from the array. If we need to only add a new element without deleting, the 2nd parameter will get in the way. (In such a use case of this method, it must be 0.)
- It is a little hard to memorize because the purpose of each parameter is not obvious. One parameter is the index of the element, another one is the quantity of elements, and then the new elements themself.
Immutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
const updatedSampleArray: Array<string> = addElementsToArray({
targetArray: experimentalSample,
newElements: [ "NEW_ELEMENT-1" ],
toPosition__numerationFrom0: 2,
mutably: false
});
console.log(sampleArray);
console.log(updatedSampleArray);
With mutably: false
the new array will be based on
the initial one, then all manipulation will be executed with the new one.
In this example, after creating the new array, the
element "NEW_ELEMENT-1"
will be added to position 2
with counting from 1.
As a result, the new array will be:
[ "INITIALLY_EXISTED_ELEMENT-1", "NEW_ELEMENT-1", "INITIALLY_EXISTED_ELEMENT-2" ]
.
However, this function does not create a full ("deep") copy of the array. First of all, it is generally impossible to create a deep copy of an arbitrary object (note: in the ECMAScript languages, the array is the superset of the object). For example, the closures and private fields added in ES2022 could not be copied, thus the function for the deep copying of an arbitrary object could not be developed. In the case of adding elements to the array, it is not critical even in React. However, in the case of changing the properties of an object-type array elements, both a new array itself and the new element based on the previous one need to be created.
Until recently, without usage of a third-party library, the same manipulation could not be done with a single expression, so it was required to:
- Create the new array
- Copy the elements to this new array
- Call the
splice
method of the new array
These routines are exactly what addElementsToArray
does when the
mutable
option has the value of false
.
In 2023, the toSpliced
method was been
developed, which does same things as splice
, but it preliminary
creates a new array based on the initial one.
Since the spring of 2023, some popular browsers do not support
the toSpliced
method, and, in Node.js,
this method has been available since version 20.
Anyway, you still need to memorize what the parameters of splice
and toSpliced
mean, so addElementsToArray
is still useful, and removal of this function from the library because of the appearance of
toSpliced
is not planned.
Adding of multiple elements to the start of array
Mutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
addElementsToArray({
targetArray: sampleArray,
newElements: [ "NEW_ELEMENT-1", "NEW_ELEMENT-2" ],
toStart: true,
mutably: true
});
console.log(sampleArray);
Adding of elements
"NEW_ELEMENT-1"
and "NEW_ELEMENT-2"
to the start of the array of
strings.
The mutably
option has been made required
not without reason; the programmer must clearly realize
what he is doing.
In this example, the value true
has been set, so the initial
array will change to:
[ "NEW_ELEMENT-1", "NEW_ELEMENT-2", "INITIALLY_EXISTED_ELEMENT-1", "INITIALLY_EXISTED_ELEMENT-2" ]
.
ReadonlyArray
because the function will change this
array.
This usage of the function is close to the native method
unshift.
The difference is that addElementsToArray
always returns
the updated array, not the count of its
elements as unshift
does.
However, if the element count of the new array is required, it is possible
to access the length
property of the
returned value without defining a new variable.
In addition, unlike unshift
, it is completely obvious
what addElementsToArray
does; specifically, whether or not adding the
elements will change the initial array.
The input required for addElementsToArray
takes more time than
unshift
; however, the crystal clearness of the code and
its maintainability are much more important than the input of the initial code.
Unfortunately, it does not convince many programmers, especially junior programmers because
human psychology prefers momentary profits over greater future profits.
In the development of web sites and software, such a feature is the cause of code that is
hard to maintain, which makes web sites and software lose time and money.
As for the input speed, modern Integrated Development Environments (IDEs)
solve such problems well.
In particular, for theIntelliJ IDEA family IDEs
theofficial YDEE plugin is supported, and it includestemplates for quick input (so-called "Live templates") , such as the one for addElementsToArray
.
Immutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
const updatedSampleArray: Array<string> = addElementsToArray({
targetArray: experimentalSample,
newElements: [ "NEW_ELEMENT-1", "NEW_ELEMENT-2" ],
toStart: true,
mutably: false
});
console.log(sampleArray);
console.log(updatedSampleArray);
In the case of immutable adding of the elements, a new
array based on the previous one will be created.
Then, the specified elements will be added to the
new one.
This way the initial array sampleArray
will
not mutate while the new one
(updatedSampleArray
) will have the value.
[ "NEW_ELEMENT-1", "NEW_ELEMENT-2", "INITIALLY_EXISTED_ELEMENT-1", "INITIALLY_EXISTED_ELEMENT-2" ]
.
Such anti-intuitive manipulations are demanded mainly in frontend frameworks, and the developers of
some of them (e. g. React) assign users with the task of creating
new state objects based on outdated ones.
addElementsToArray
allows you to write clearer code, in particular when you
need to add one or more elements to the intermediate positions of the
array.
This makes the code simpler.
However, this function does not create a full ("deep") copy of the array. First of all, it is generally impossible to create a deep copy of an arbitrary object (note: in the ECMAScript languages, the array is the superset of the object). For example, the closures and private fields added in ES2022 could not be copied, thus the function for the deep copying of an arbitrary object could not be developed. In the case of adding elements to the array, it is not critical even in React. However, in the case of changing the properties of an object-type array elements, both a new array itself and the new element based on the previous one need to be created.
Adding of multiple elements to the end of array
Mutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
addElementsToArray({
targetArray: sampleArray,
newElements: [ "NEW_ELEMENT-1", "NEW_ELEMENT-2" ],
toEnd: true,
mutably: true
});
console.log(sampleArray);
Adding of elements
"NEW_ELEMENT-1"
and "NEW_ELEMENT-2"
to the end of the array of
strings.
The mutably
option has been made required
not without reason; the programmer must clearly realize
what he is doing.
In this example, the value true
has been set, so the initial
array will change to:
[ "INITIALLY_EXISTED_ELEMENT-1", "INITIALLY_EXISTED_ELEMENT-2", "NEW_ELEMENT-1", "NEW_ELEMENT-2" ]
.
ReadonlyArray
because the function will change this
array.
This usage of the function is close to the native method
push.
The difference is that addElementsToArray
always returns
the updated array, not the count of its
elements as push
does.
However, if the element count of the new array is required, it is possible
to access the length
property of the
returned value without defining a new variable.
In addition, unlike push
, it is completely obvious
what addElementsToArray
does; specifically, whether or not adding the
elements will change the initial array.
The input required for addElementsToArray
takes more time than
push
; however, the crystal clearness of the code and
its maintainability are much more important than the input of the initial code.
Unfortunately, it does not convince many programmers, especially junior programmers because
human psychology prefers momentary profits over greater future profits.
In the development of web sites and software, such a feature is the cause of code that is
hard to maintain, which makes web sites and software lose time and money.
As for the input speed, modern Integrated Development Environments (IDEs)
solve such problems well.
In particular, for theIntelliJ IDEA family IDEs
theofficial YDEE plugin is supported, and it includestemplates for quick input (so-called "Live templates") , such as the one for addElementsToArray
.
Immutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
const updatedSampleArray: Array<string> = addElementsToArray({
targetArray: experimentalSample,
newElements: [ "NEW_ELEMENT-1", "NEW_ELEMENT-2" ],
toEnd: true,
mutably: false
});
console.log(sampleArray);
console.log(updatedSampleArray);
In the case of immutable adding of the elements, a new
array based on the previous one will be created.
Then, the specified elements will be added to the
new one.
This way the initial array sampleArray
will
not mutate while the new one
(updatedSampleArray
) will have the value.
[ "INITIALLY_EXISTED_ELEMENT-1", "INITIALLY_EXISTED_ELEMENT-2", "NEW_ELEMENT-1", "NEW_ELEMENT-2" ]
.
Such anti-intuitive manipulations are demanded mainly in frontend frameworks, and the developers of
some of them (e. g. React) assign users with the task of creating
new state objects based on outdated ones.
addElementsToArray
allows you to write clearer code, in particular when you
need to add one or more elements to the intermediate positions of the
array.
This makes the code simpler.
However, this function does not create a full ("deep") copy of the array. First of all, it is generally impossible to create a deep copy of an arbitrary object (note: in the ECMAScript languages, the array is the superset of the object). For example, the closures and private fields added in ES2022 could not be copied, thus the function for the deep copying of an arbitrary object could not be developed. In the case of adding elements to the array, it is not critical even in React. However, in the case of changing the properties of an object-type array elements, both a new array itself and the new element based on the previous one need to be created.
Adding of multiple elements to specific position (numeration from 0)
Mutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
addElementsToArray({
targetArray: experimentalSample,
newElements: [ "NEW_ELEMENT-1", "NEW_ELEMENT-2" ],
toPosition__numerationFrom0: 1,
mutably: true
});
console.log(sampleArray);
Adding the new
elements "NEW_ELEMENT-1"
and
"NEW_ELEMENT-2"
to 1st position
(counting from 0).
Because the initial array had 2 elements,
the new elements will be between them, and the
updated array will be:
[ "INITIALLY_EXISTED_ELEMENT-1", "NEW_ELEMENT-1", "NEW_ELEMENT-2", "INITIALLY_EXISTED_ELEMENT-2" ]
.
The same thing could be done without libraries by using the native method splice, but:
- This method was designed primarily for deleting elements from the array. If we need to only add a new element without deleting, the 2nd parameter will get in the way. (In such a use case of this method, it must be 0.)
- It is a little hard to memorize because the purpose of each parameter is not obvious. One parameter is the index of the element, another one is the quantity of elements, and then the new elements themself.
Immutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
const updatedSampleArray: Array<string> = addElementsToArray({
targetArray: experimentalSample,
newElements: [ "NEW_ELEMENT-1", "NEW_ELEMENT-2" ],
toPosition__numerationFrom0: 1,
mutably: false
});
console.log(sampleArray);
With mutably: false
the new array will be based on
the initial one, then all manipulation will be executed with the new one.
In this example, after creating the new array, the
elements "NEW_ELEMENT-1"
and
"NEW_ELEMENT-2"
will be added to position 1
with counting from 0.
As a result, the new array will be:
[ "INITIALLY_EXISTED_ELEMENT-1", "NEW_ELEMENT-1", "NEW_ELEMENT-2", "INITIALLY_EXISTED_ELEMENT-2" ]
.
However, this function does not create a full ("deep") copy of the array. First of all, it is generally impossible to create a deep copy of an arbitrary object (note: in the ECMAScript languages, the array is the superset of the object). For example, the closures and private fields added in ES2022 could not be copied, thus the function for the deep copying of an arbitrary object could not be developed. In the case of adding elements to the array, it is not critical even in React. However, in the case of changing the properties of an object-type array elements, both a new array itself and the new element based on the previous one need to be created.
Until recently, without usage of a third-party library, the same manipulation could not be done with a single expression, so it was required to:
- Create the new array
- Copy the elements to this new array
- Call the
splice
method of the new array
These routines are exactly what addElementsToArray
does when the
mutable
option has the value of false
.
In 2023, the toSpliced
method was been
developed, which does same things as splice
, but it preliminary
creates a new array based on the initial one.
Since the spring of 2023, some popular browsers do not support
the toSpliced
method, and, in Node.js,
this method has been available since version 20.
Anyway, you still need to memorize what the parameters of splice
and toSpliced
mean, so addElementsToArray
is still useful, and removal of this function from the library because of the appearance of
toSpliced
is not planned.
Adding of multiple elements to specific position (numeration from 1)
Mutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
addElementsToArray({
targetArray: experimentalSample,
newElements: [ "NEW_ELEMENT-1", "NEW_ELEMENT-2" ],
toPosition__numerationFrom0: 2,
mutably: true
});
console.log(sampleArray);
Adding the new
elements "NEW_ELEMENT-1"
and
"NEW_ELEMENT-2"
to 2nd position
(counting from 1).
Because the initial array had 2 elements,
the new elements will be between them, and the
updated array will be:
[ "INITIALLY_EXISTED_ELEMENT-1", "NEW_ELEMENT-1", "NEW_ELEMENT-2", "INITIALLY_EXISTED_ELEMENT-2" ]
.
The same thing could be done without libraries by using the native method splice, but:
- This method was designed primarily for deleting elements from the array. If we need to only add a new element without deleting, the 2nd parameter will get in the way. (In such a use case of this method, it must be 0.)
- It is a little hard to memorize because the purpose of each parameter is not obvious. One parameter is the index of the element, another one is the quantity of elements, and then the new elements themself.
Immutable adding
const sampleArray: Array<string> = [
"INITIALLY_EXISTED_ELEMENT-1",
"INITIALLY_EXISTED_ELEMENT-2"
];
const updatedSampleArray: Array<string> = addElementsToArray({
targetArray: experimentalSample,
newElements: [ "NEW_ELEMENT-1", "NEW_ELEMENT-2" ],
toPosition__numerationFrom0: 1,
mutably: false
});
console.log(sampleArray);
With mutably: false
the new array will be based on
the initial one, then all manipulation will be executed with the new one.
In this example, after creating the new array, the
elements "NEW_ELEMENT-1"
and
"NEW_ELEMENT-2"
will be added to position 2
with counting from 1.
As a result, the new array will be:
[ "INITIALLY_EXISTED_ELEMENT-1", "NEW_ELEMENT-1", "NEW_ELEMENT-2", "INITIALLY_EXISTED_ELEMENT-2" ]
.
However, this function does not create a full ("deep") copy of the array. First of all, it is generally impossible to create a deep copy of an arbitrary object (note: in the ECMAScript languages, the array is the superset of the object). For example, the closures and private fields added in ES2022 could not be copied, thus the function for the deep copying of an arbitrary object could not be developed. In the case of adding elements to the array, it is not critical even in React. However, in the case of changing the properties of an object-type array elements, both a new array itself and the new element based on the previous one need to be created.
Until recently, without usage of a third-party library, the same manipulation could not be done with a single expression, so it was required to:
- Create the new array
- Copy the elements to this new array
- Call the
splice
method of the new array
These routines are exactly what addElementsToArray
does when the
mutable
option has the value of false
.
In 2023, the toSpliced
method was been
developed, which does same things as splice
, but it preliminary
creates a new array based on the initial one.
Since the spring of 2023, some popular browsers do not support
the toSpliced
method, and, in Node.js,
this method has been available since version 20.
Anyway, you still need to memorize what the parameters of splice
and toSpliced
mean, so addElementsToArray
is still useful, and removal of this function from the library because of the appearance of
toSpliced
is not planned.
Quick input in IntelliJ IDEA family of IDEs
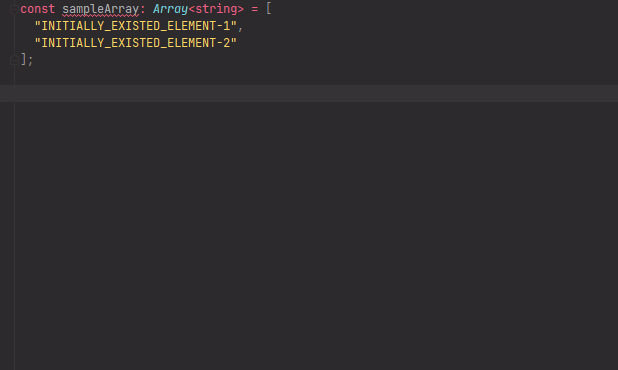
Using the functionality of Live templates in the IntelliJ IDEA family if IDEs allows you to quickly input code such as a function invocation expression. To get the Live templates of the YDEE library, you need to install the official plugin of this library.
Steps for using the Live templates
If you have not used Live templates before, do not worry if the instructions below are too complicated. Once you have developed the habit of using Live templates (similar to the habit of using keyboard shortcuts), the following operations will take a matter of seconds.
- Copy the variable name containing the array or array expression to the clipboard. To make it possible for the IDE to fill in the correct value for the parameter, please develop the habit of copying each time before inputting the Live template of the addElementsToArray function.
- Begin to input the function name (addElementsToArray).
There will be 2 options for autocomplete:
- Circled icon with the letter: it is the autocompletion of the function name, which is the standard functionality of the IDE. If you press the Enter key, the full function name will be inputted and also the function import declaration will be inserted if required. Not bad, but better automation is possible.
- The icon with the cliche is the template we need.
Press Enter again.
The code template will be inserted with the value of the
targetArray
property filled with the clipboard content, and then it is selected by the cursor. If you follow this manual, you don’t need to edit the inserted value, so exit thetargetArray
property editing mode by pressing Enter again.
- Type the value of
newElements
. Once finished, press Enter. If the unwanted (in this case) autocomplete interferes with your work, press Esc first. - You will be suggested to select the value of the mutably property from
the dropdown list.
The
true
value is pre-selected. If you need this, proceed to the next step by the pressing Enter. Otherwise, using the down arrow key, select optionfalse
first. - You will be suggested to input the value of the
toPosition__numerationFrom0
property. Once inputted, the value oftoPosition__numerationFrom1
will be filled in as well. However, at least one of these two must be deleted. If you need one of these options, input the numeric value of the name of the desired variable. Anyway, once done, pressEnter
. - Delete the unnecessary code.
Among
toStart
,toEnd
,toPosition__numerationFrom0
andtoPosition__numerationFrom1
only one must remain.
You can use the alias of this Live Template — aeta
,
which consists of the first letters of all words of the function name.
However, the disadvantage of such aliases is that they are harder to memorize, while all you need to do to
memorize the autocomplete of addElementsToArray is to remember the beginning
of the name of the function.